Logic programming
Program = Facts + Rules or
Program = Logic + Control
Different paradigms
Logic programming
Program = Facts + Rules or
Program = Logic + Control
Imperative programming
Program = Data structures + Algorithms
Logic programming: first steps
1969 PLANNER programming language (MIT, by Carl Hewitt)
1970 SHRDLU (MIT, by Terry Winograd): NL conversation program about block world
Person: Which cube is sitting on the table?
Computer: THE LARGE GREEN ONE WHICH SUPPORTS THE RED PYRAMID.
Person: Is there a large block behind a pyramid?
Computer: YES, THREE OF THEM: A LARGE RED ONE, A LARGE GREEN CUBE, AND THE BLUE ONE.
Person: Put a small one onto the green cube which supports a pyramid.
Computer: OK.
Logic programming: Prolog
1972 Prolog (Marseille, by Alain Colmerauer et al.)
enables programmers to write highly declarative programs that express intuitively logical queries,
uses a very powerful backtracking algorithm to answer those queries.
Prolog language basic syntax (1/3)
variables (named or anonymous, like last one) X, Value, A12, _42, Res, _
numbers 1, -15, 3.1415, 0.23e-5
constants (AKA atoms) aa, [], 'hello', @
logical connectives: ,and, ;or
comments: % full line or /* C-style inline */
Prolog language basic syntax (2/3)
compound terms:
can denote nested data structures person(name(curt), age(27))
can denote predicates (logical relations, coming next) and functions (relations between expressions and values, stay tuned)
Name of the compound term is called a functor.
To refer to a term we can write its functor and the number of arguments: person/2, age/1etc.
Prolog language basic syntax (3/3)
facts (named relations between entities in the problem domain)
female(elizabeth).
% 'elizabeth' is a parent of 'charles'
parent(elisabeth, charles). % here ',' is just a comma
rules (named relations that can be inferred from other relations)
% X is a mother of Y IF X is a parent of Y AND X is female
mother(X, Y) :- parent(X, Y), female(X). % here ',' is a connective
Prolog programs are sets of clauses, that can be either.
Horn clauses
Prolog clauses H :- B1, ..., Bn.
are instances of Horn clausesH if B0 and B1 and ... and Bn
H is the head and B0 and B0 and ... Bn is the body of the clause.
HEAD BODY
mother(X,Y) IF parent(X,Y) AND female(X) % rule
female(X) IF true % fact
Computation in an interpreter
Prolog program execution starts with a query (AKA goal)
marked as ?- in the interpreter window ->
A query is a statement that can be answered by expansion using facs and rules.
Running example
Let's consider the domain of genealogical relationships in the British Royal family, written as relations:
facts: female/1, male/1, parent/2
rules: mother/2, father/2
Closed world assumption
Anything that cannot be inferred with given data is assumed false.
Here this is illustrated for the query ?- female(meghan).
Computation mechanisms
Queries are answered by:
matching (sub)goals against facts or rules
unifying free variables with terms (NOT assignment)
backtracking when (sub)goal matching fails
Let's briefly see these three mechanisms on the family tree example.
Lists consist of either an empty list[], or a non-empty list (e.g.[a | [b,c]]) consisting of the head element (a) and the tail of the list ([b,c]). Free variables are allowed.
Pattern Matching in Lists
Consider predicate for checking list membership
in(X, [X | _ ]). in(X, [ _ | L]) :- in(X, L).
and several different queries:
No Order
Since there is no notion of input and return arguments, any argument can be both:
Have this for extended slides
---
### Roadmap (still may change)
- Logic programming: why, when, how
- Prolog facts and rules
- SLD resolution: Searching and Backtracking
- Lists and Terms
- Functions, Recursion, Arithmetics
- Extensions (briefly)
--
## Logic programming in perspective
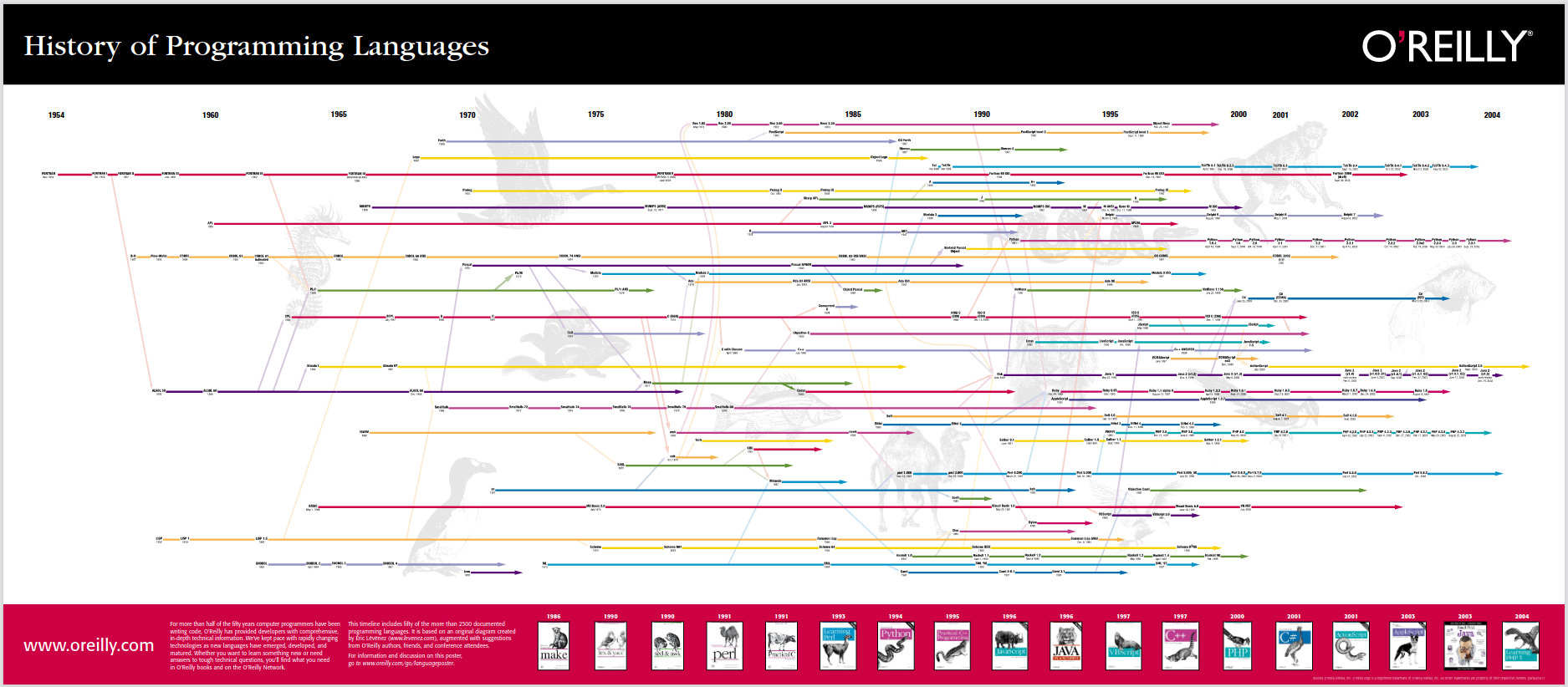
full version of the poster in [here](https://cdn.oreillystatic.com/news/graphics/prog_lang_poster.pdf)
not covered: strings
```prolog
female(elisabeth). parent(elisabeth, charles). % R1
mother(X,Y) :- parent(X, Y), female(X). parent(elisabeth, anne). % R2
```
rule matching with variable unification for `?- mother(elisabeth, Y)`
```prolog
parent(elisabeth, Y) , female(elisabeth) % X = elisabeth, Y free
parent(elisabeth, charles), true % Y = charles (from R1)
```
possible backtracking on subgoal `parent(elisabeth, Y)`:
```prolog
parent(elisabeth, Y) , female(elisabeth) % X = elisabeth, Y free
parent(elisabeth, anne), true % Y = anne (from R2)
```
can be exercise on comparing clause and literal order